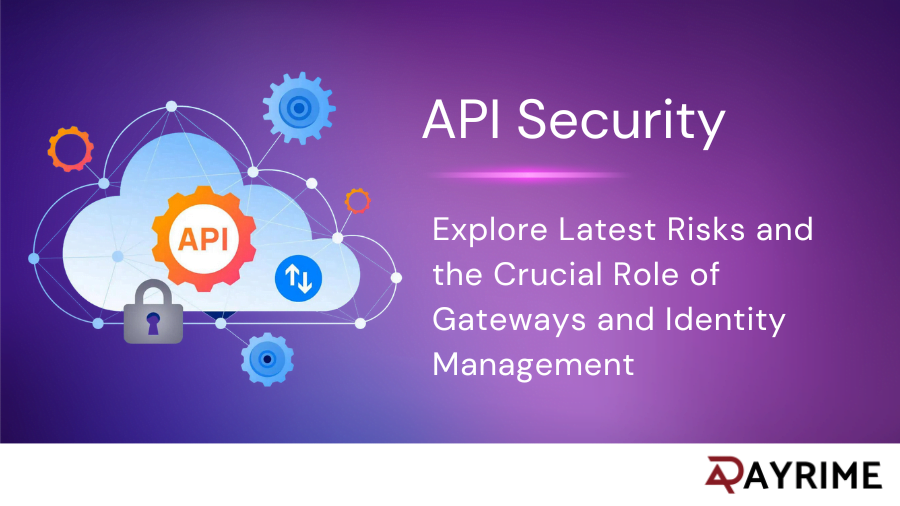
Introduction
A vulnerable API can serve as a gateway for data leaks, system intrusions, and various security nightmares. Crafting a solid API security strategy is crucial, extending beyond the mere prevention of misuse. It’s about preserving your data integrity, maintaining your brand’s trustworthiness, achieving compliance standards, and cementing trust with your clientele and business allies.
Throughout this piece, we delve into essential API security concerns, delving into areas from stringent authentication methods to robust encryption practices. These elements are key to reinforcing the security of your API infrastructure.
Plus, stay tuned for our strategic insights on holistic API security to shield your operations from fraudulent activities.
Top 10 API Security Risks in 2023 and Mitigation Strategies
1. Broken Object Level Authorization
APIs frequently expose endpoints that deal with object identifiers, which could cause Object Level Access Control problems and pave the way for attackers.
In other words, occurs in a scenario where a user is able to access data that they shouldn’t have access to due to insufficient authorization checks.
For instance, consider a social media platform with an API endpoint that allows a user to fetch messages by providing a message ID.
If the API doesn’t properly verify that the user requesting the message is actually authorized to see it (e.g., by checking if the user is part of the conversation), then it could allow the user to access any message just by changing the message ID in the request.
This would constitute a broken object-level authorization security issue.
To mitigate Broken Object Level Authorization, the following strategies can be employed:
- Use a Centralized Access Control: Implement a robust, centralized access control mechanism that checks the user’s permissions before accessing any object.
- Least Privilege Principle: Grant users the minimum level of access (or permissions) necessary to perform their required functions.
- Validation and Verification: Always validate and verify the user’s access rights on the server side before providing access to any object based on its identifier.
- Audit Logs: Keep detailed audit logs that record who accessed what objects and when.
- Regular Access Reviews: Conduct frequent reviews of access control policies and user rights to ensure they are up-to-date and secure.
- Automated Testing: Utilize automated tools to test for authorization checks on each endpoint and object.
2. Broken Authentication
In this risk, attackers may utilize poorly built authentication mechanisms to assume the identity of users.
This can involve scenarios where an attacker is able to compromise authentication tokens or exploit flaws in the authentication process.
This could happen, for instance, if an application does not properly validate the authenticity of session tokens or if the tokens are easily guessable due to weak generation algorithms.
If an attacker gains access to a user’s session token, they can impersonate that user and perform unauthorized actions within the application.
This vulnerability may also arise from using default credentials, inadequate password recovery mechanisms, or failure to implement multi-factor authentication.
Mitigating Broken Authentication requires a multifaceted approach:
- Implement Strong Authentication Mechanisms: Use multi-factor authentication and strong, adaptive authentication mechanisms.
- Secure Password Storage: Store passwords using a strong, adaptive, and salted hashing algorithm.
- Token Security: Ensure tokens are securely generated, stored, and invalidated after logout, inactivity, or expiration.
- Encryption: Use HTTPS to encrypt data in transit.
- Monitor and Log Authentication Attempts: Log and monitor failed login attempts to detect and prevent brute-force attacks.
- Session Management: Create secure session management that includes secure token generation, expiration, and invalidation strategies.
- API Throttling: Use throttling mechanisms to protect against automated attacks.
- Automated Testing: Perform automated security and vulnerability testing on authentication features.
- Security Headers and Policies: Utilize security headers and policies such as Content Security Policy (CSP) to protect against cross-site scripting (XSS) and related attacks.
- Up-to-date Libraries: Keep all libraries and frameworks up to date to prevent known vulnerabilities
3. Broken Object Property Level Authorization
This risk originates from insufficient authorization validation at the object property level, which is a mix of Excessive Data Exposure and Mass Assignment.
An example of Broken Object Property Level Authorization would be when an API endpoint allows a user to update their profile information, such as their email address and password.
Suppose the API endpoint does not properly validate the properties being updated and does not check whether the user is authorized to change each property.
An attacker could potentially send a request that includes properties not intended to be exposed to the user, such as changing the user’s account role from a regular user to an administrator.
Here’s a hypothetical API request that illustrates this:
PATCH /api/users/123
Content-Type: application/json
Authorization: Bearer user-token
{
\"email\": \"user@example.com\",
\"password\": \"newpassword\",
\"role\": \"admin\" // The attacker adds a property that they shouldn't have access to modify
}
In this example, if the API does not check whether the user is permitted to change the \”role\” property, and processes the request, it would result in unauthorized privilege escalation. This is why proper authorization checks must be in place at the object property level to prevent such security issues.
Mitigation:
To correct the issue of Broken Object Property Level Authorization in the example given, you would need to implement server-side checks that verify whether the user making the request has the authority to update each specific property. Here’s how you could address the problem:
- Validate Input: Ensure that the API only accepts properties that should be editable by the user. Any unexpected properties should be rejected.
- Verify Permissions: Before applying any changes, the API should check the user’s permissions for each property they are attempting to update. If a user is not authorized to modify a property, the request should be denied.
- Limit Access: Implement role-based access control (RBAC) or attribute-based access control (ABAC) to define what properties each role or attribute can modify.
Here is a pseudocode representation of what the server-side check might look like:
def update_user_profile(user_id, updates):
user = get_user_by_id(user_id)
if not user:
return \"User not found\"
for property, value in updates.items():
if not user.has_permission_to_update(property):
return f\"Unauthorized to update {property}\"
user.apply_updates(updates)
return \"Profile updated successfully\"
In this function, has_permission_to_update
would be a method that checks whether the current user has the appropriate permissions to update a given property. If they don\’t, the function returns an unauthorized message for that property.
4. Unrestricted Resource Consumption
Refers to a situation where an API does not properly manage the allocation of system resources, like CPU, memory, network bandwidth, or disk space, for each user or service. This can lead to vulnerabilities where an attacker might perform actions that consume excessive amounts of resources, causing a denial of service (DoS) for legitimate users or driving up operational costs by exploiting paid resources like SMS or biometric validation services.
An example of Unrestricted Resource Consumption would be an API endpoint that allows file uploads without restrictions on the size or number of files that can be uploaded. An attacker could exploit this by uploading very large files or a large number of files, thereby consuming server storage space, exhausting system memory, or overloading the network bandwidth.
Python using Flask to create an API endpoint that corrects unrestricted resource consumption by imposing file size limits and rate limiting:
from flask import Flask, request, jsonify
from flask_limiter import Limiter
from flask_limiter.util import get_remote_address
app = Flask(__name__)
# Configure a basic in-memory rate limiter
limiter = Limiter(
app,
key_func=get_remote_address,
default_limits=[\"10 per minute\"] # Rate limiting to 10 requests per minute
)
MAX_FILE_SIZE = 1024 * 1024 * 2 # 2 MB limit
@app.route(\'/upload\', methods=[\'POST\'])
@limiter.limit(\"5 per minute\") # More granular rate limit for this endpoint
def upload_file():
file = request.files[\'file\']
if file:
# Check if the file size is too large
if file.content_length > MAX_FILE_SIZE:
return jsonify({\"error\": \"File size exceeds limit\"}), 413
# Here you would save the file, and process it
# save_file(file)
return jsonify({\"message\": \"File uploaded successfully\"}), 200
else:
return jsonify({\"error\": \"No file provided\"}), 400
if __name__ == \'__main__\':
app.run(debug=True)
This would prevent an attacker from uploading excessively large files or bombarding the server with too many requests in a short period, thereby mitigating unrestricted resource consumption.
To correct this, the API should:
- Implement Rate Limiting: Limit the number of requests a user can make within a certain timeframe.
- Enforce Quotas: Set a maximum storage limit per user or per upload.
- Validate Input: Check the file types and sizes before processing them.
- Monitor Usage: Keep an eye on usage patterns and set up alerts for unusual activity.
- Efficient Resource Allocation: Use streaming, chunking, or other techniques to process large files without requiring significant system resources.
- Use a WAF: Employ a Web Application Firewall to block malicious traffic patterns.
- Circuit Breaker Pattern: Implement fail-safes that cut off resource-heavy operations if they reach a certain threshold.
Additionally, monitoring and automated alerting systems can be used to detect and respond to abnormal usage patterns indicative of an attack.
5. Broken Function Level Authorization
Broken Function Level Authorization occurs when users are able to execute functions for which they do not have permission. This can happen due to improper enforcement of access controls on various functions within an API.
For example, a user with regular permissions might be able to access administrative functions due to a misconfiguration or oversight in the access control logic.
showcasing a simple API with function-level authorization checks:
from flask import Flask, request, jsonify, abort
from functools import wraps
app = Flask(__name__)
# Mock database of users with roles
users_db = {
\"alice\": {\"password\": \"alicepass\", \"role\": \"admin\"},
\"bob\": {\"password\": \"bobpass\", \"role\": \"user\"}
}
# Mock function to check user credentials
def check_credentials(username, password):
user = users_db.get(username)
return user and user[\'password\'] == password
# Decorator for requiring admin role to access a function
def require_admin(f):
@wraps(f)
def decorated_function(*args, **kwargs):
auth = request.authorization
if not auth or not check_credentials(auth.username, auth.password) \\
or users_db[auth.username][\'role\'] != \'admin\':
abort(403)
return f(*args, **kwargs)
return decorated_function
@app.route(\'/admin/data\')
@require_admin
def admin_data():
return jsonify({\"admin\": \"data\"})
if __name__ == \'__main__\':
app.run(debug=True)
In this code:
- We define a simple user database with roles.
- We have a decorator
require_admin
that wraps around an endpoint to check if the authenticated user is an admin. - The
/admin/data
endpoint is protected by therequire_admin
decorator, so only users with the admin role can access it.
If a regular user tries to access the /admin/data
endpoint, they will receive a 403 Forbidden
response, thus preventing broken function level authorization.
To correct this, it’s crucial to:
- Implement strong access control checks that are enforced on every function.
- Ensure that changes in user roles are reflected immediately in their access rights.
- Regularly audit and test access controls to ensure they are functioning as expected.
6. Unrestricted Access to Sensitive Business Flows
APIs that expose business flows without taking into account harmful overuse can be troublesome.
Unrestricted Access to Sensitive Business Flows refers to the lack of adequate protection around business processes that are exposed via APIs, such as payment transactions, order processing, or user registration. If these flows aren’t properly secured, attackers can exploit them, for instance, by automating fraudulent transactions or spamming an order system.
To correct this:
- Implement strict rate limiting and transaction controls.
- Use CAPTCHAs to deter automated access.
- Require strong authentication and authorization checks before allowing access to sensitive business operations.
7. Server-Side Request Forgery
This risk occurs when an API retrieves a remote resource without validating the user-supplied URI
Server-side request Forgery (SSRF) is a security vulnerability that occurs when a server-side application is tricked into making a request to an unintended location. In an SSRF attack, the attacker can make the application send a request to internal services within the organization\’s infrastructure or to external third-party systems, potentially bypassing firewalls and other security measures.
Here’s a code example that demonstrates an API endpoint in Python using Flask, which includes mitigations against SSRF:
from flask import Flask, request, jsonify
import requests
from urllib.parse import urlparse
app = Flask(__name__)
ALLOWED_DOMAINS = {\"example.com\", \"api.example.com\"}
@app.route(\'/fetch-data\')
def fetch_data():
url = request.args.get(\'url\', \'\')
parsed_url = urlparse(url)
# Validate the URL hostname is in the allowed list
if parsed_url.hostname not in ALLOWED_DOMAINS:
return jsonify({\"error\": \"Invalid URL provided\"}), 400
# Make the HTTP request to the external service
response = requests.get(url)
# Return the response content
return jsonify({\"data\": response.text})
if __name__ == \'__main__\':
app.run(debug=True)
In this example, an allowlist of domains ensures that only predefined domains can be requested, mitigating the risk of SSRF attacks. The urlparse
function is used to validate the hostname of the URL provided by the user. If the URL is not in the allowed list, the request is rejected.
To protect against SSRF:
- Validate and sanitize user inputs to ensure they do not contain malicious URLs.
- Implement strict allowlists for the destinations that the server can request.
- Ensure that server-to-server communication is authenticated and encrypted where possible.
- Use a server-side proxy with a strict allowlist to handle outgoing requests.
8. Security Misconfiguration
Complex configurations can cause errors that pave the way for attacks.
Security Misconfiguration occurs when security settings are defined, implemented, or maintained incorrectly, often leading to unnecessary vulnerabilities. This can include misconfigured HTTP headers, overly detailed error messages, unnecessary services running on the server, default accounts with unchanged passwords, and outdated software with known vulnerabilities.
For example, a web server that has directory listing enabled by default can expose files and directories to an attacker. The flaw in the Nginx server configuration might look like this:
server {
listen 80;
server_name example.com;
# ... other configuration ...
# Directory listing is enabled by default
location / {
autoindex on; # Flaw: Directory listing is enabled
# ... rest of the configuration ...
}
# ... other configuration ...
}
Here, autoindex on;
enables directory listing, which could inadvertently expose sensitive files and directories to users who navigate to a URL path on the server that doesn\’t have an index file. Correcting this flaw involves setting autoindex off;
to disable directory listing and protect the contents of directories from being listed and accessed by unauthorized users.
Here’s how you might address this issue:
server {
listen 80;
server_name example.com;
# ... other configuration ...
# Disable directory listing
location / {
autoindex off;
# ... rest of the configuration ...
}
# ... other configuration ...
}
In this Nginx configuration example, autoindex off;
is used to disable directory listing for the entire server. This prevents clients from seeing a list of files in a directory when no index file (like index.html) is present.
To correct this:
- Regularly update and patch systems and applications.
- Follow a hardening guide to properly configure servers and software.
- Remove or disable unnecessary features and services.
- Use automated scanners to detect and report misconfigurations.
- Ensure proper error handling to prevent the leak of sensitive information.
- Change default logins and passwords.
- Limit and monitor access to configuration settings.
9. Improper Inventory Management
Improper Inventory Management in the context of API security refers to the insufficient tracking and documentation of API endpoints and versions. This can lead to outdated or deprecated APIs remaining in production, which may not have the latest security patches or features, and can also lead to accidental exposure of endpoints meant for internal use or debugging.
To correct this:
- Maintain an accurate inventory of all active APIs and their versions.
- Ensure that documentation is updated, reflecting the current API landscape.
- Use automated tools to detect and catalog endpoints.
- Regularly review and decommission unused or outdated APIs.
10. Unsafe Consumption of APIs
Unsafe Consumption of APIs refers to the practice of trusting and integrating third-party APIs without applying the same level of security scrutiny as to internal APIs. This can create vulnerabilities if the third-party services are compromised or if they don’t adhere to strict security standards.
To correct this, you should:
- Validate all data from third-party APIs as if they were user input.
- Enforce strict timeout and error handling for third-party API calls to avoid system failures.
- Regularly review the security posture of third-party services.
- Use API gateways to monitor and potentially sanitize the data passing between third-party services and your system.
The Role of API Gateways in API Security
In today’s digital world, API security is crucial, and API gateways play a crucial part in API security.
What is an API Gateway?
An API gateway serves as an intermediate between clients and backend services and is crucial for maintaining API security. It accepts and routes API requests while standardizing the communication flow to increase security.
Why Use API Gateways?
API gateways provide a structured approach to large-scale API management by taking care of routine activities like user authentication and rate limits. They separate client interactions from backend activities, making it easier for clients to deal with sophisticated backend procedures.
Key Features of API Gateways
- Security: Controls authorization as well as authentication.
- Access: Responsible for quotas and throttling.
- Routing and Caching: Includes cache management and potentially processes to an internal API.
- Monitoring: Ensures the best possible API performance.
- Versioning: Occasionally involves automation.
Advantages and Drawbacks of Gateways
Advantages:
- Centralized
- Simplified
- Consistently managed policy
Drawbacks:
- Single-Point of Failure
- Complexity risks
- Migration Difficulties
The Role of Identity Management in API Security
Identity management is crucial for API security since it ensures that only authorized users can access applications. Identity in the modern day increasingly includes factors like location, behavior, and timing patterns in addition to merely a username and password. It’s critical to combine identity with behavioral analytics and anomaly detection for the best API security, rather than just relying on identity alone. It is recommended to follow the zero-trust strategy, which requires verification of each API access regardless of the source.
Tokens are important in the world of API security. Bearer tokens are widely used, although they can be insecure if they are stolen. Proof-of-possession (PoP) tokens provide a higher level of security since they demand client verification. To avoid data inconsistencies, it is also crucial to guarantee consistent user identification across different authentication methods. The authentication process determines an identity’s level of trust, and decisions about API access should be based heavily on these trust levels. As a result, identity management is essential in establishing how API risks and potential data breaches can be reduced through the implementation of suitable authentication and authorization protocols.
Conclusion
In conclusion, securing APIs is an essential task that transcends mere best practices—it requires a culture of security mindfulness that pervades every level of an organization. From the initial stages of API design to the post-deployment phase, it is vital to remain vigilant and proactive in identifying and mitigating risks.